Programming In C Practical 13
Question 1
Find sum of digits of a given number using do-while loop.
Sum.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num,rem,sum=0;
printf("\nEnter a number: ");
scanf("%d",&num);
do{
rem=num%10;
sum=sum+rem;
num=num/10;
}
while(num>0);
printf("\nSum of digits is: %d",sum);
getch();
}
Output

Question 2
Write a program to reverse the number of given number print using while loop
Reverse.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num,rem,rev=0;
printf("\nEnter a number: ");
scanf("%d",&num);
while(num>0)
{
rem=num%10;
rev=rev*10+rem;
num=num/10;
}
printf("\nReverse of given number is: %d",rev);
getch();
}
Output
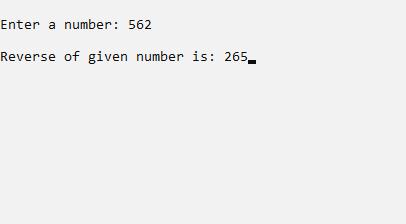
Question 3
Write a program to check given number is palindrome or not using while loop.
Palindrom.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num,rem,temp,rev=0;
printf("\nEnter a number: ");
scanf("%d",&num);
temp=num;
while(num>0)
{
rem=num%10;
rev=rev*10+rem;
num=num/10;
}
if(temp==rev)
printf("\nNumber is palindrom");
else
printf("\nNumber is not palindrom");
getch();
}
Output
