Mobile Application Development Practical 12
Question 1
Write a program to show the following output. First two radio buttons are without using radio group and next two radio buttons are using radio group. Note the changes between these two and also toast which radio button is selected.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_gravity="center_horizontal"
android:textSize="20dp"
android:text="Single Radio Buttons" />
<RadioButton
android:id="@+id/radioButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="Radio Button 1" />
<RadioButton
android:id="@+id/radioButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="Radio Button 2" />
<View
android:id="@+id/divider"
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="?android:attr/listDivider"
android:layout_marginTop="10dp"/>
<RadioGroup
android:id="@+id/rgp"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<RadioButton
android:id="@+id/rbmale"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="Male" />
<RadioButton
android:id="@+id/rbfemale"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="Female" />
</RadioGroup>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:background="@color/black"
android:backgroundTint="#7C7A7A"
android:text="Show selected"
/>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.practical12;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
RadioButton r1,r2,r;
RadioGroup rg;
Button b;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
r1=findViewById(R.id.radioButton1);
r2=findViewById(R.id.radioButton2);
rg=findViewById(R.id.rgp);
b=findViewById(R.id.button);
View.OnClickListener cl=new View.OnClickListener() {
@Override
public void onClick(View view) {
String msg="";
if(r1.isChecked())
msg=msg+"Radio Button 1";
if(r2.isChecked())
msg=msg+",Radio Button 2";
if(!msg.equals(""))
Toast.makeText(MainActivity.this,"Selected radio button: "+msg,Toast.LENGTH_LONG).show();
int rid=rg.getCheckedRadioButtonId();
r=findViewById(rid);
if(r!=null)
Toast.makeText(MainActivity.this,"Selected Gender:"+r.getText().toString(),Toast.LENGTH_LONG).show();
}
};
b.setOnClickListener(cl);
}
}
Output
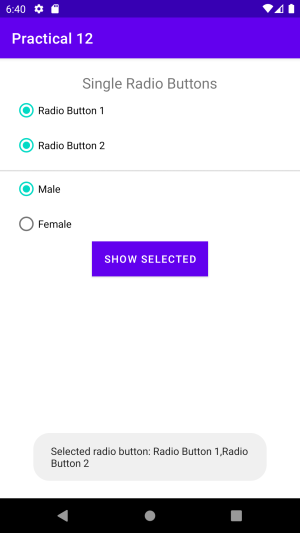
