Object Oriented Programming Practical 18
Question 1
Write a C++ Program to interchange the values of two int , float and char using function overloading.
swap.cpp
C
#include<iostream.h>
#include<conio.h>
void swap(int a,int b)
{
int temp=a;
a=b;
b=temp;
cout<<"\nAfter swapping number1 is "<<a<<" and number2 is "<<b<<endl;
}
void swap(float a,float b)
{
float temp=a;
a=b;
b=temp;
cout<<"\nAfter swapping float1 is "<<a<<" and float2 is "<<b<<endl;
}
void swap(char a,char b)
{
char temp=a;
a=b;
b=temp;
cout<<"\nAfter swapping character1 is "<<a<<" and character2 is "<<b<<endl;
}
void main()
{
clrscr();
int num1,num2;
float f1,f2;
char c1,c2;
cout<<"\nEnter two integers: ";
cin>>num1>>num2;
cout<<"\nEnter two float numbers: ";
cin>>f1>>f2;
cout<<"\nEnter two characters: ";
cin>>c1>>c2;
swap(num1,num2);
swap(f1,f2);
swap(c1,c2);
getch();
}
Output

Question 2
Write a C++ Program that find the sum of two int and double number using function overloading.
sum.cpp
C
#include<iostream.h>
#include<conio.h>
int sum(int a, int b)
{
return a + b;
}
double sum(double a, double b)
{
return a + b;
}
int main()
{
int int1,int2;
double double1,double2;
cout<<"\nEnter two integers: ";
cin>>int1>>int2;
cout<<"\nEnter two double values: ";
cin>>double1>>double2;
int intSum = sum(int1, int2);
double doubleSum = sum(double1, double2);
cout << "\nSum of " << int1 << " and " << int2 << " is: " << intSum << endl;
cout << "Sum of " << double1 << " and " << double2 << " is: " << doubleSum << endl;
getch();
return 0;
}
Output
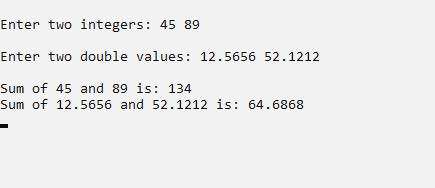
Question 3
Write C++ program to find the area of various geometrical shapes by Function overloading.
shape.cpp
C
#include<iostream.h>
#include<conio.h>
class shape
{
public:
void area(int x)
{
int area=x*x;
cout<<"\nArea of square is: "<<area<<endl;
}
void area(int x,int y)
{
int area=x*y;
cout<<"\nArea of rectangle is: "<<area<<endl;
}
};
void main()
{
clrscr();
int side1,side2;
shape s;
cout<<"\nEnter side1: ";
cin>>side1;
cout<<"\nEnter side2: ";
cin>>side2;
s.area(side1);
s.area(side1,side2);
getch();
}
Output
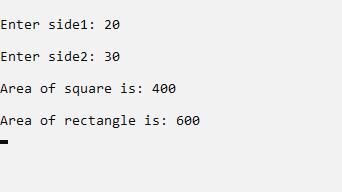