Advanced Java Programming Practical 15
Question 1
Write a program using URL class to retrieve the host, protocol, port and file of URL http://www.msbte.org.in
URLDemo.java
Java
import java.net.*;
class URLDemo
{
public static void main(String args[])throws MalformedURLException
{
URL netAddress=new URL("http://www.msbte.org.in");
System.out.println("Protocol:"+netAddress.getProtocol());
System.out.println("Port:"+netAddress.getPort());
System.out.println("Host:"+netAddress.getHost());
System.out.println("File:"+netAddress.getFile());
}
}
Output
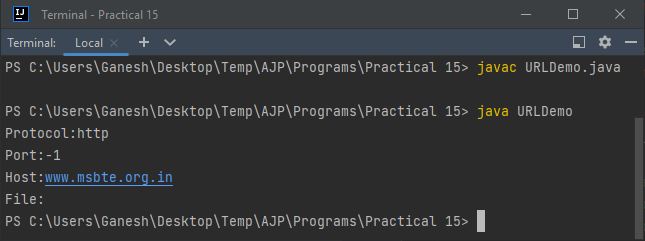
Question 2
Write a program using URL and URLConnection class to retrieve the date, content type, content length information of any entered URL
URLData.java
Java
import java.io.*;
import java.net.*;
import java.util.*;
public class URLData
{
public static void main(String[] args) throws IOException, MalformedURLException
{
DataInputStream din = new DataInputStream(System.in);
System.out.print("Enter an Url: ");
String u = din.readLine();
URL url = new URL(u);
URLConnection uc = url.openConnection();
System.out.println("Date:"+ new Date(uc.getDate()) );
System.out.println("Content Type: "+ uc.getContentType());
System.out.println("Content Length: "+ uc.getContentLength());
}
}
Output
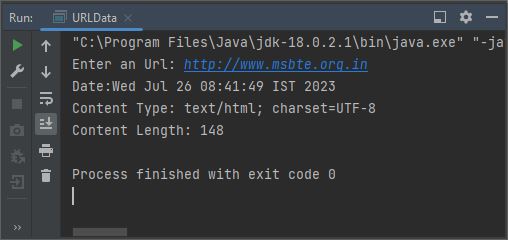