Advanced Java Programming Practical 18
Question 1
Develop a program to create employee table in database having two columns “emp_id” and “emp_name”.
DBDemo.java
Java
import java.sql.*;
class DBDemo
{
public static void main(String args[])
{
try
{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Driver loaded");
Connection con=DriverManager.getConnection("jdbc:mysql://localhost:3306/javadb1","root","");
System.out.println("Connection to the database created");
Statement st = con.createStatement();
String query = "create table employee(emp_id int(10),emp_name varchar(20))";
st.execute(query);
System.out.println("Table created");
}
catch (SQLException e)
{
System.out.println(e);
}
catch (ClassNotFoundException e)
{
throw new RuntimeException(e);
}
}
}
Output
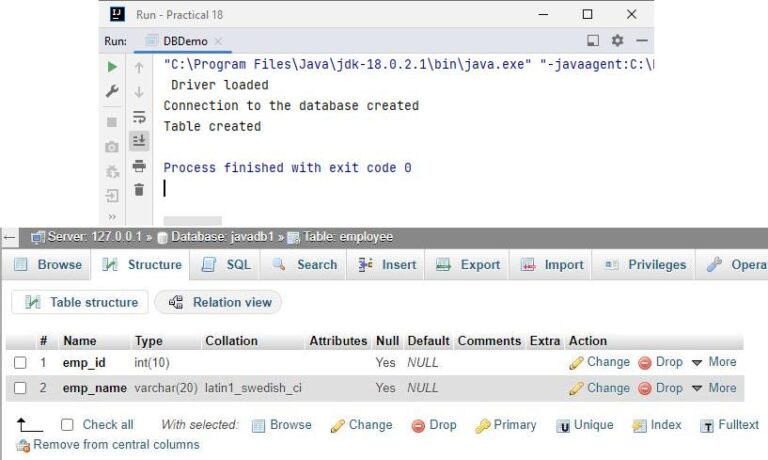
Question 2
Develop a program to display the name and roll_no of students from “student table” having percentage > 70.
PercentDemo.java
Java
import java.sql.*;
public class PercentDemo
{
public static void main(String args[])
{
try
{
Class.forName("com.mysql.jdbc.Driver");
System.out.println("Driver loaded");
Connection con= DriverManager.getConnection("jdbc:mysql://localhost:3306/javadb1","root","");
System.out.println("Connection to the database created");
Statement st = con.createStatement();
String query = "select * from student where percent > 70";
ResultSet rs=st.executeQuery(query);
String text="";
System.out.println("Students with more than 70 percent are:");
System.out.println("Roll Number\t\t\tName\t\t\t\tPercent");
while(rs.next())
{
System.out.println(rs.getInt(1)+"\t\t\t"+rs.getString(2)+"\t\t\t"+rs.getFloat(3));
}
}
catch (SQLException e)
{
System.out.println(e);
}
catch (ClassNotFoundException e)
{
throw new RuntimeException(e);
}
}
}
Output
