Advanced Java Programming Practical 24
Question 1
Develop a program to collect user information using cookie.
UserCookie.java
Java
import java.io.*;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class UserCookie extends HttpServlet
{
public void doPost(HttpServletRequest request,HttpServletResponse response)throws ServletException,IOException
{
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>User information</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>User information using cookie</h1>");
String uname=request.getParameter("uname");
String password=request.getParameter("pass");
Cookie c1=new Cookie("user",uname);
Cookie c2=new Cookie("pass",password);
response.addCookie(c1);
response.addCookie(c2);
out.println("<h3>Cookie Name : "+c1.getName()+"<br>");
out.println("Cookie Value : "+c1.getValue()+"<br>");
out.println("Cookie Name : "+c2.getName()+"<br>");
out.println("Cookie Value : "+c2.getValue()+"<br>");
out.println("</h3></body></html>");
out.close();
}
}
Output
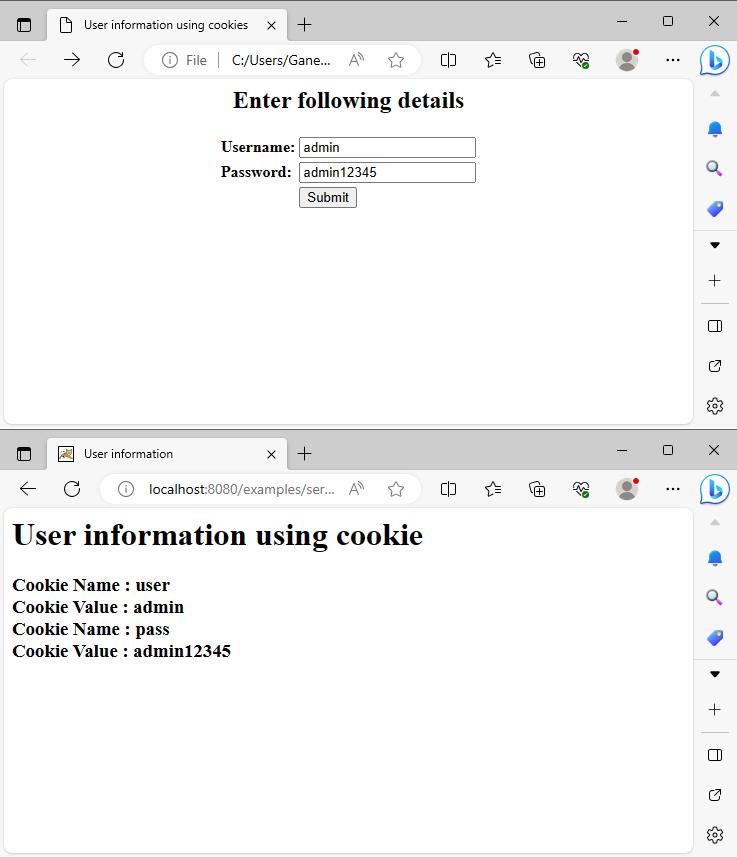
Question 2
Develop program to get the browser Information.
BrowserDetails.java
Java
import java.io.*;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class BrowserDetails extends HttpServlet
{
public void doGet(HttpServletRequest request,HttpServletResponse response)throws ServletException,IOException
{
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>Browser information</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Browser information</h1>");
String browser = request.getHeader("user-agent");
out.println("<h4>The Web-Browser you are using is : ");
if(browser.indexOf("MSIE")!=-1)
out.println("Microsoft Internet Explorer");
else if(browser.indexOf("Chrome")!=-1)
out.println("Google Chrome");
else if(browser.indexOf("Firefox")!=-1)
out.println("Mozilla Firefox");
else
out.println("You are using any other browser</h4>");
out.println("</body></html>");
out.close();
}
}
Output
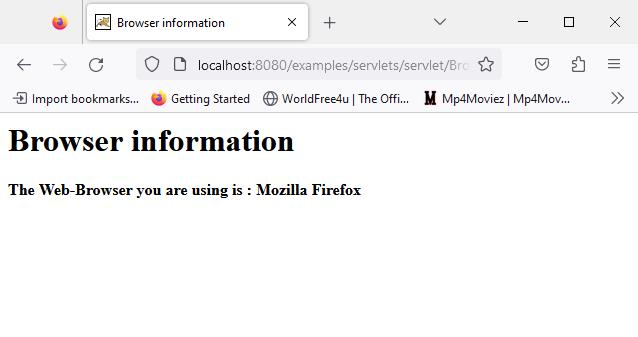