Mobile Application Development Practical 6
Question 1
Write a program to display 10 students basic information in a table form using Table layout.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TableLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="Roll No" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Student Name" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3301" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Mahesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3302" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Suresh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3303" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Ramesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3304" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Dinesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3305" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Nilesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3306" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Haresh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3307" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Nagesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3308" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Ratnesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3309" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Prathamesh" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginLeft="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="3310" />
<TextView
android:id="@+id/textView"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:text="Nikesh" />
</TableRow>
</TableLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.practical6;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
Output
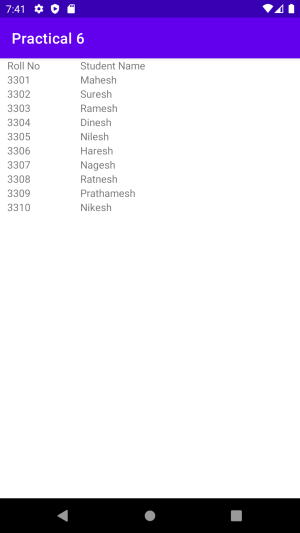
Question 2
Write a program to display all the data types in object-oriented programming using Frame layout.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Integer"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Character"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Float"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Double"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Class"/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Array"/>
</LinearLayout>
</FrameLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.practical6_1;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
Output
