Object Oriented Programming Practical 20
Question 1
Write a C++ program to add two complex numbers using operator overloading by a friend function.
complex.cpp
C
#include<iostream.h>
#include<conio.h>
class complex
{
int real,img;
public:
void accept()
{
cout<<"\nEnter real part of complex number: ";
cin>>real;
cout<<"\nEnter imaginary part of complex number: ";
cin>>img;
}
void display()
{
cout<<"\nReal part of complex number= "<<real;
cout<<"\nImaginary part of complex number= "<<img<<endl;
}
friend complex operator+(complex,complex);
};
complex operator+(complex x,complex y)
{
complex c;
c.real=x.real+y.real;
c.img=x.img+y.img;
return c;
}
void main()
{
clrscr();
complex c1,c2,c3;
cout<<"\nComplex number 1";
c1.accept();
cout<<"\nComplex number 2";
c2.accept();
c3=c1+c2;
cout<<"\nComplex number 3";
c3.display();
getch();
}
Output

Question 2
Write a C++ program to subtract two complex numbers using operator overloading by using member function.
complex2.cpp
C
#include<iostream.h>
#include<conio.h>
class complex
{
int real,img;
public:
void accept()
{
cout<<"\nEnter real part of complex number: ";
cin>>real;
cout<<"\nEnter imaginary part of complex number: ";
cin>>img;
}
void display()
{
cout<<"\nReal part of complex number= "<<real;
cout<<"\nImaginary part of complex number= "<<img<<endl;
}
complex operator-(complex x)
{
complex c;
c.real=x.real+real;
c.img=x.img+img;
return c;
}
};
void main()
{
clrscr();
complex c1,c2,c3;
cout<<"\nComplex number 1";
c1.accept();
cout<<"\nComplex number 2";
c2.accept();
c3=c1-c2;
cout<<"\nComplex number 3";
c3.display();
getch();
}
Output
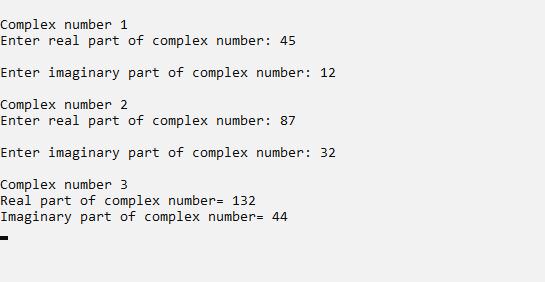
Question 3
Write a C++ program to compare two strings using'==' operator overloading.
compare.cpp
C
#include<iostream.h>
#include<conio.h>
class compare
{
char str[50];
public:
void accept()
{
cout<<"\nEnter string: ";
cin>>str;
}
void operator==(compare x)
{
int c=strcmp(str,x.str);
if(c==0)
cout<<"\nBoth strings are equal";
else
cout<<"\nBoth strings are not equal";
}
};
void main()
{
clrscr();
compare c1,c2;
cout<<"\nString 1";
c1.accept();
cout<<"\nString 2";
c2.accept();
c1==c2;
getch();
}
Output
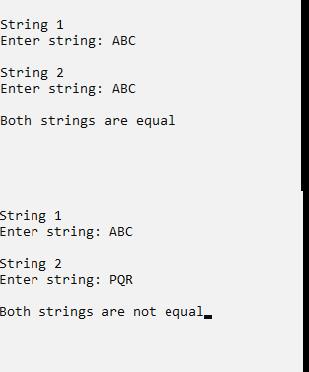