Object Oriented Programming Practical 21
Question 1
Define a class parent in which use read function, define another class child use same read function. Display the data of both the read function on output screen using virtual function.
virtual.cpp
C
#include<iostream.h>
#include<conio.h>
class Parent
{
public:
virtual void read()
{
cout << "Parent class read function" << endl;
}
};
class Child : public Parent
{
public:
void read()
{
cout << "Child class read function" << endl;
}
};
int main()
{
Parent *ptr;
Parent p;
Child c;
ptr = &p;
ptr->read();
ptr = &c;
ptr->read();
getch();
return 0;
}
Output
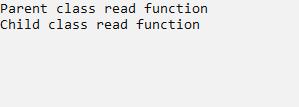
Question 2
Write a program which shows the use of function overriding.
override.cpp
C
#include<iostream.h>
#include<conio.h>
class Base
{
public:
virtual void display()
{
cout << "Display function in Base class" << endl;
}
};
class Derived : public Base
{
public:
void display()
{
cout << "Display function in Derived class" << endl;
}
};
int main()
{
Base baseObj;
Derived derivedObj;
baseObj.display();
derivedObj.display();
getch();
return 0;
}
Output
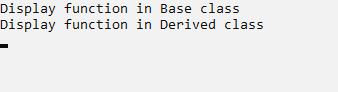