Object Oriented Programming Practical 26
Question 1
Write a C++ program to copy entire content of one binary file at a time to another file using read () and write ()
binary.cpp
C
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream inFile;
ofstream outFile;
inFile.open("input.bin", ios::binary);
if (!inFile)
{
cout << "Error: Unable to open input file!" << endl;
return 1;
}
outFile.open("output.bin", ios::binary);
if (!outFile) {
cout << "Error: Unable to open output file!" << endl;
inFile.close();
return 1;
}
char buffer[1024];
while (inFile.read(buffer, sizeof(buffer)))
{
outFile.write(buffer, inFile.gcount());
}
outFile.write(buffer, inFile.gcount());
inFile.close();
outFile.close();
cout << "Binary file copied successfully!" << endl;
return 0;
}
Output
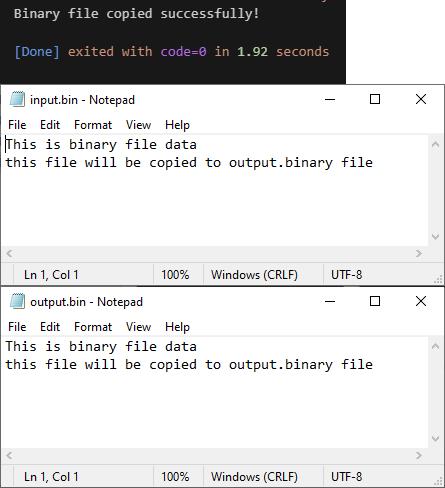