Programming In C Practical 19
Question 1
Create a structure called a library to hold details of the book viz. accession number, title of the book, author name, price of the book and flag indicating whether book is issued or not. Fetch some sample data and display the same.
Library.cpp
C
#include<stdio.h>
#include<conio.h>
#include<string.h>
struct library
{
int acssno,flag;
float price;
char title[50],author[50];
}l;
void main()
{
clrscr();
printf("\nEnter title of a book: ");
gets(l.title);
printf("\nEnter author of a book: ");
gets(l.author);
printf("\nEnter access number of a book: ");
scanf("%d",&l.acssno);
printf("\nEnter price of a book: ");
scanf("%f",&l.price);
printf("\nEnter book issued or not(1:yes/0:no): ");
scanf("%d",&l.flag);
printf("\n\n---------Book details---------- ");
printf("\nTitle of a book: %s",l.title);
printf("\nAuthor of a book: %s",l.author);
printf("\nAccess number of a book: %d",l.acssno);
printf("\nPrice of a book: rupees %f",l.price);
if(l.flag==1)
printf("\nBook is issued");
else
printf("\nBook is not issued");
getch();
}
Output
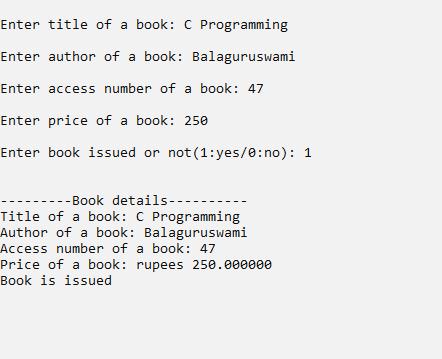
Question 2
Develop and execute C program to Add Two distances given in kilometer-meter using structures.
Distance.cpp
C
#include<stdio.h>
#include<conio.h>
#include<string.h>
struct distance
{
int km,m;
}d1,d2,d3;
void main()
{
clrscr();
printf("\nEnter kilometers of distance 1: ");
scanf("%d",&d1.km);
printf("\nEnter meters of distance 1: ");
scanf("%d",&d1.m);
printf("\nEnter kilometers of distance 2: ");
scanf("%d",&d2.km);
printf("\nEnter meters of distance 2: ");
scanf("%d",&d2.m);
d3.m=d1.m+d2.m;
d3.km=d1.km+d2.km+d3.m/1000;
d3.m=d3.m%1000;
printf("\nAddition of two distances is: %d kilometers and %d meters",d3.km,d3.m);
getch();
}
Output
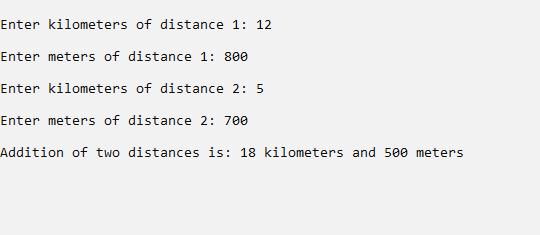