Programming In C Practical 5
Question 1
Write a C program to read integer, character, float, double values from the user and display it. Mention different format specifiers used in program in comment.
values.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int i;
char ch;
float f;
double d;
printf("\nEnter an integer: ");
scanf("%d",&i);
printf("\nEnter a character: ");
scanf(" %c",&ch);
printf("\nEnter a float value: ");
scanf("%f",&f);
printf("\nEnter a double value: ");
scanf("%lf",&d);
printf("\nYour values are");
printf("\nInteger value: %d",i);
printf("\nCharacter value: %c",ch);
printf("\nFloat value: %f",f);
printf("\nDouble value: %lf",d);
getch();
}
Output

Question 2
Write a C program to print the address of variable. mention format specifier used in comment.
address.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num;
printf("\nEnter a number: ");
scanf("%d",&num);
printf("\nAddress of variable num is: %u",&num);
getch();
}
Output
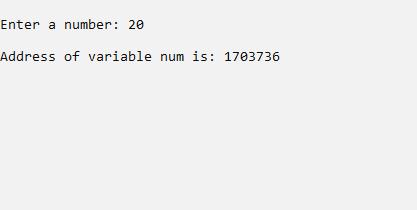