Programming In C Practical 6
Question 1
Write a program for the largest of three numbers.
Largest.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num1,num2,num3;
printf("\nEnter first number: ");
scanf("%d",&num1);
printf("\nEnter second number: ");
scanf("%d",&num2);
printf("\nEnter third number: ");
scanf("%d",&num3);
if(num1>num2)
{
if(num1>num3)
printf("\n%d is largest",num1);
}
if(num2>num1)
{
if(num2>num3)
printf("\n%d is largest",num2);
}
if(num3>num1)
{
if(num3>num2)
printf("\n%d is largest",num3);
}
getch();
}
Output
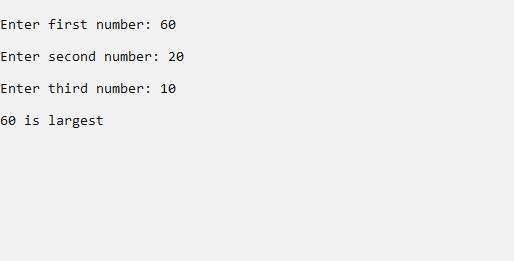
Question 2
Write a program to demonstrate the working of relational operator.
Evenodd.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num;
printf("\nEnter a number : ");
scanf("%d",&num);
if(num%2==0)
printf("\nNumber is even number");
else
printf("\nNumber is odd number");
getch();
}
Output

Question 3
Write a program using conditional operator to check whether a user can vote or not based on their age.
Vote.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int age;
printf("\nEnter an age of a person: ");
scanf("%d",&age);
(age>=18)?printf("\nPerson is eligible to vote"):printf("\nPerson is not eligible to vote");
getch();
}
Output
