Programming In C Practical 7
Question 1
Write a program to find the greatest number between three by using logical operators.
Largest.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num1,num2,num3;
printf("\nEnter first number: ");
scanf("%d",&num1);
printf("\nEnter second number: ");
scanf("%d",&num2);
printf("\nEnter third number: ");
scanf("%d",&num3);
if(num1>num2&&num1>num3)
{
printf("\n%d is largest",num1);
}
if(num2>num1&&num2>num3)
{
printf("\n%d is largest",num2);
}
if(num3>num1&&num3>num2)
{
printf("\n%d is largest",num3);
}
getch();
}
Output

Question 2
Write a program that accepts marks of 5 subjects calculate the percentage and display grade of student from percentage. (Grade Distinction, first class, second class, pass class, fail).
Grade.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int mark1,mark2,mark3,mark4,mark5,total;
float percent;
printf("\nEnter marks of first subject: ");
scanf("%d",&mark1);
printf("\nEnter marks of second subject: ");
scanf("%d",&mark2);
printf("\nEnter marks of third subject: ");
scanf("%d",&mark3);
printf("\nEnter marks of forth subject: ");
scanf("%d",&mark4);
printf("\nEnter marks of fifth subject: ");
scanf("%d",&mark5);
total=mark1+mark2+mark3+mark4+mark5;
percent=(total*100)/500;
if(percent>=75&&percent<=100)
{
printf("\nYour grade is: Distinction");
}
if(percent>=60&&percent<75)
{
printf("\nYour grade is: First class");
}
if(percent>=50&&percent<60)
{
printf("\nYour grade is: Second class");
}
if(percent>=40&&percent<50)
{
printf("\nYour grade is: Pass class");
}
if(percent>=0&&percent<40)
{
printf("\nYour grade is: Fail");
}
getch();
}
Output
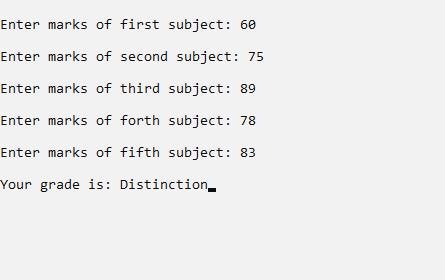
Question 3
Write a program to find whether the given number is even and divisible by 5 or not.
Number.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int num;
printf("\nEnter a number : ");
scanf("%d",&num);
if(num%2==0&&num%5==0)
printf("\nNumber is even and divisible by 5");
else
printf("\nNumber is odd or not divisible by 5");
getch();
}
Output
