Programming In C Practical 9
Question 1
Write a C program to determine whether a given year is a leap year or not.
Leapyear.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int year;
printf("\nEnter an year: ");
scanf("%d",&year);
if(year%4==0)
{
printf("\nYear is leap year");
}
else
{
printf("\nYear is not a leap year");
}
getch();
}
Output
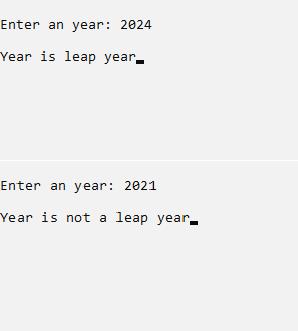
Question 2
Write a C program to determine whether a string is palindrome.
Palindrom.cpp
C
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
char str[30];
int i=0,j=0,flag=0;
printf("\nEnter a String: ");
scanf("%s",str);
while(str[j]!='\0')
j++;
j--;
while(str[i]!='\0')
{
if(str[i]!=str[j])
flag=1;
i++;
j--;
}
if(flag==0)
{
printf("\nString is palindrom");
}
else
{
printf("\nString is not palindrom");
}
getch();
}
Output
